直播基础功能 Android接入方法
一、获取Feed流
使用场景
有一个完整的页面可以展示直播feed,需要一下无限loading的直播feed。
示意图
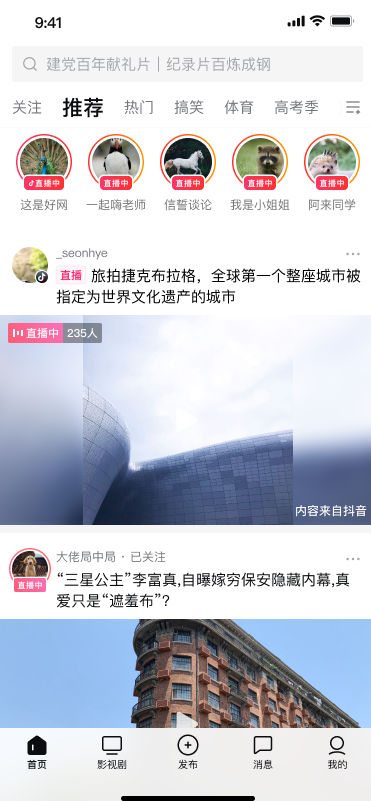
接入方式
以下方式可获取单列、方形大卡样式的列表。TTLiveService.``_getLiveService_
().getOpenLiveListFactory().createLiveFragment(params
)
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// ......
FragmentTransaction ft = getSupportFragmentManager().beginTransaction();
// 1. 初始化参数
OpenFeedParams params = new OpenFeedParams.Builder(FeedType.COMMON_FEED)
.feedStyle(FeedStyle.SINGLE_LIST_H)
.setEnableFeedTitle(true)
.setEnableFeedBackIcon(true)
.build();
// 2. 获取直播feed列表
Fragment feedFragment = TTLiveService.getLiveService().getOpenLiveListFact
ory().createLiveFragment(params);
ft.add(R.id.fragment_container, feedFragment);
ft.commit();
}
参数说明
OpenFeedParams,以下配置为Feed列表所有配置项,部分配置项只针对特定
FeedStyle生效,具体可参考后续表格
OpenFeedParams feedPrams = new OpenFeedParams.Builder(feedType) // feed 类
型,关注feed/推荐feed
.feedStyle(feedStyle) // feed样式,目前仅支持SINGLE_LIST_H
.supportFollowListInFeed(true) // 是否显示关注天窗,默认不显示
.setEnableFeedTitle(true) // 是否显示title bar
.setFeedTitle("抖音直播") // 配置直播feedtitle
.setEnableFeedBackIcon(true) // 是否显示返回按钮,当title bar显示时生效
.setFeedBackIcon(newBackDrawable) // 配置title bar返回按钮,支持传入Drawable
.setVerticalFeedItemDecoration(dp2px(4)) // 配置卡片垂直方向的间距,仅双列样式生效
.setHorizontalFeedItemDecoration(dp2px(4)) // 配置卡片水平方向的间距,单双列样式都生效
.setEnableOperationLabel(false) // 是否显示活动标签
.setEnableContentLabel(false) // 是否显示内容标签 (PK,红包,聊天室等标签)
.setLiveTagColor(Color.BLACK) // 当feed样式有直播中状态标签时,支持配置标签背景色
.setLiveTagRadius(dp2px(2)) // 当feed样式有直播中状态标签时,支持配置标签圆角
.build();
FeedStyle (1.8.3版本支持SINGLE_LIST_H, 其余样式1.8.4版本支持)
enum class FeedStyle {
DOUBLE_LIST_D, // 双列列表,方形小卡片
DOUBLE_LIST_X, // 双列列表,长方形小卡片
SIMPLE_LIST, // 单列列表,类似关注列表
SINGLE_LIST_X, // 单列列表,长方形大卡片,可预览
SINGLE_LIST_H // 单列列表,方形大卡片,可预览
}
卡片样式对应的配置项
卡片样式 | FeedStyle | 支持的配置项 | 配置项方法调用 |
单列-方形 大卡+预览流 | FeedStyle.SINGLE _LIST_X | 1.配置列表间距 2.配置标签有无 | 1. setHorizontalFeedItemDecoration(dp2px(4)) 2. setEnableOperationLabel(false) setEnableContentLabel(false) |
单列-长方形 大卡+预览流 | SINGLE_LIST_X | 1.配置标签有无 2.配置直播中标签 背景色,圆角 | 1. setEnableOperationLabel(false) setEnableContentLabel(false) 2. setLiveTagColor(Color.BLACK) setLiveTagRadius(dp2px(2)) |
双列-方形卡片 (无预览流) | DOUBLE_LIST_D | 1.配置标签有无 2.配置列表横向, 纵向间距 3.配置卡片圆角 | 1. setEnableOperationLabel(false) setEnableContentLabel(false) 2. setHorizontalFeedItemDecoration(dp2px(4)) setVerticalFeedItemDecoration(dp2px(4)) 3. setFeedCardCornerRadius(dp2Px(6f)) |
双列-长方形 卡片 (无预览流) | DOUBLE_LIST_X | 1. 配置标签有无 2. 配置列表横向, 纵向间距 | 1. setEnableOperationLabel(false) setEnableContentLabel(false) 2. setHorizontalFeedItemDecoration(dp2px(4)) setVerticalFeedItemDecoration(dp2px(4)) |
单列-简单列表 (类似关注列表) | SIMPLE_LIST | 1. 配置标签有无 2. 配置直播中标 签背景色, 3. 呼吸圈颜色 | 1. setEnableOperationLabel(false) setEnableContentLabel(false) 2. setLiveTagColor(Color.BLACK) |
二、Feed卡片
2.1 获取卡片
使用场景
接入方有自己的feed,想在自己的feed流中插入几个直播卡片,UI和数据源均由直播提供;
目前仅提供预览流样式的封面卡片,对应CardStyle.PREVIEW_CARD_H(1.8.3版
本),CardStyle.PREVIEW_CARD_X(1.8.4版本)
示意图
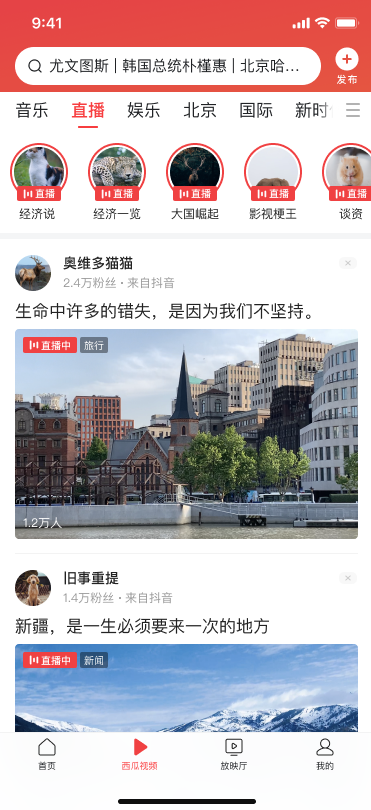
接入方式
- 接口定义
ILiveListManager:用于获取cardView列表
ILiveCardView:卡片的抽象接口,返回的ILiveCardView都是View,可以添加到父布局中。
// ILiveListManager: 用于获取card view列表
interface ILiveListManager {
/**
* @param context
* @param openFeedParams feed参数
* @return 异步获取 List<ILiveCardView>
*/
fun requestAndGetView(context: Context, openFeedParams: OpenFeedPar
ams) : Observable<List<ILiveCardView>>
}
// 直播封面卡片接口
interface ILiveCardView {
fun bindRoom(room: Room, enterFromMerge: String?, liveCoverConfig: LiveCoverCo
nfig?) // 可选,如果外部提供数据结
构,则调用该接口绑定数据
/**
* 是否支持播放预览流
*/
fun enablePreview(): Boolean
/**
* view展示的时候调用,用于上报埋点
*/
fun onShow()
/**
* 播放预览流
*/
fun startPreview()
/**
* 停止播放
*/
fun stopPreview(destroyPlayer: Boolean)
}
- 使用示例
通过ILiveListManager#requestAndGetView
直接获取view。
ILiveCardView可能具有预览功能,使用步骤如下:
- 调用
enablePreview()
判断是否支持预览。 - 根据滑动位置停留位置获取对应位置的ILiveCardView,调用
startPreview()
- 播放预览流。
- 根据滑动位置暂停预览流,调用
stopPreview()
暂停预览流。建议在整个页面 - 退出的时候调用
stopPreview(true)
销毁播放器实例,具体时机参考下面代码示例。
注意:直播sdk仅提供ILiveCardView,滑动控制逻辑需要接入方自行实现,由于卡片内部复用
播放器,同一时间只能播放一个预览流,在调用startPreview之前请确保已经将
上一个正在播放的预览流暂停。
public class LiveFeedActivity extends Activity{
// 1. 获取factory
private val liveListFactory: IOpenLiveListFactory? by lazy {
TTLiveService.getLiveService()?.openLiveListFactory
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activty_feed_test)
liveListFactory?.let {
// 2. 配置参数
val hsCardParams = OpenFeedParams.Builder(FeedType.COMMON_FEED)
.setCardCount(2)
.setCardStyle(CardStyle.PREVIEW_CARD_H)
.build()
hsCardListManager = it.createLiveCardManager(this)
// 3. 通过ILiveListManager请求数据且返回card view
hsCardListManager?.requestAndGetView(this, hsCardParams)
?.subscribeOn(AndroidSchedulers.mainThread())
?.subscribe({ list ->
// 展示2个直播大卡
if (list?.isNotEmpty() == true) {
val size = 2.coerceAtMost(list.size)
for (i in 0 until size) {
hs_large_card_container.addView(list[i] as View)
hsCardList.add(list[i])
// 4.1 显示的时候调用onShow()上报埋点
list[i].onShow()
}
if (hsCardList?.isNotEmpty()) {
hs_large_card_container.post {
previewIndex = 0
previewNeedRelease = true
releaseIndex = 0
// 4.2 调用startPreview显示预览流
hsCardList[0].startPreview()
}
}
}
}, { error ->
error.printStackTrace()
})
}
}
@Override
protected void onResume() {
super.onResume();
// 6. 如果退出页面时暂停了预览流,再回到当前页面尝试重新播放预览流
if (stopPreviewWhenResume && cardList.size() > 0) {
cardList.get(previewIndex).startPreview();
}
}
@Override
protected void onPause() {
super.onPause();
// 5. 在页面onPause时暂停预览流,节约内存资源
if (previewIndex > -1) {
stopPreviewWhenResume = true;
cardList.get(previewIndex).stopPreview(false);
}
}
@Override
protected void onDestroy() {
super.onDestroy();
// 7. 页面销毁时暂停预览流,并释放播放器
if (previewNeedRelease && releaseIndex > -1) {
cardList.get(releaseIndex).stopPreview(true);
}
}
}
参数说明
val hsCardParams = OpenFeedParams.Builder(FeedType.COMMON_FEED) // feed 类
型,关注feed/推荐feed
.setCardCount(2) // 卡片个数
.setCardStyle(CardStyle.PREVIEW_CARD_H) // 卡片样式,1.8.3版本支持PREVIE
W_CARD_H,1.8.4版本支持
PREVIEW_CARD_X
.build()
2.2 双排卡片
使用场景
希望页面中既有直播卡片,又有宿主自己的元素,可通过获取一部分直播列表添
加到页面中。目前仅支持
FeedStyle.DOUBLE_LIST_D。
示意图
双列正方形(model.style = IESLiveSaaSFeedShowStyleSquDouble)
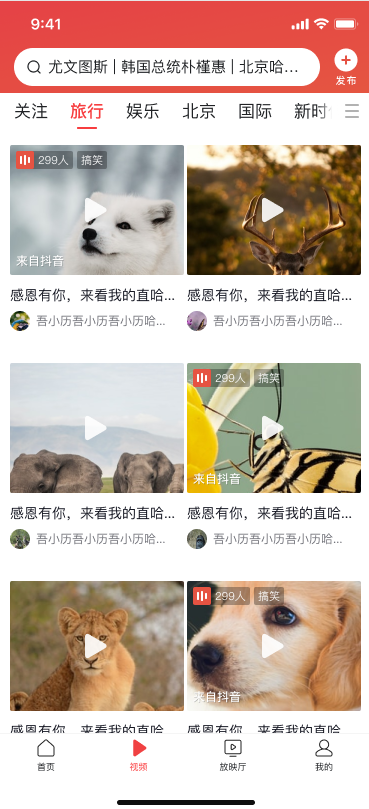
双列长方形(model.style = IESLiveSaaSFeedShowStyleRecDouble)
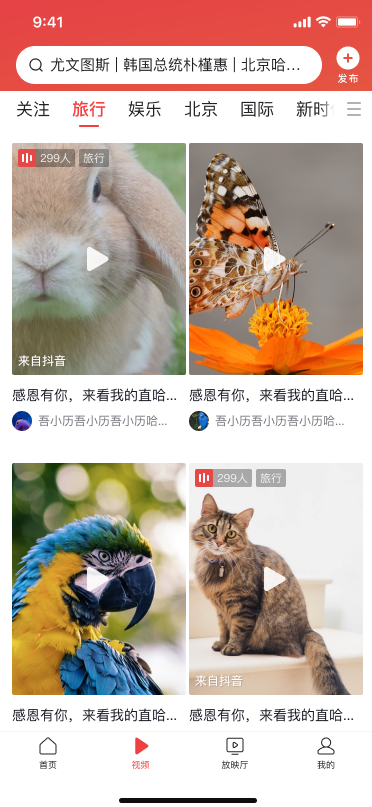
接入方式
// LiveFeedActivity
private void addFeedBlock(Intent intent) {
// 1. 通过TTLiveService获取feed服务
IOpenLiveListFactory feedFactory = TTLiveService.getLiveService().g
etOpenLiveListFactory();
if (feedFactory != null) {
// 2. 配置参数
OpenFeedParams params = new OpenFeedParams.BlockParamsBuilder()
.feedStyle(FeedStyle.DOUBLE_LIST_D)
.setEnableMoreLiveEntry(true) // 是否显示更多直播入口,默认显示
.setFeedTitle("抖音直播") // 配置直播标题
.build();
// 3. 获取模块block,这是一个view,添加到container中,并设置布局参数
ILiveBlock feedBlock = feedFactory.createLiveBlock(this, params);
FrameLayout container = findViewById(R.id.container);
container.removeAllViews();
FrameLayout.LayoutParams lp = new FrameLayout.LayoutParams(View
Group.LayoutParams.
MATCH_PARENT, ViewGroup.LayoutParams.WRAP_CONTENT);
lp.gravity = Gravity.TOP;
container.addView((View) feedBlock, lp);
// 4. 调用refresh()请求数据,同时监听列表为空和列表请求错误的状态,显示相应提示
feedBlock.refresh();
feedBlock.isEmpty().observe(this, isEmpty -> {
if (isEmpty) {
Toast.makeText(this, "暂无数据", Toast.LENGTH_SHORT).show();
}
});
feedBlock.isError().observe(this, isError -> {
if (isError) {
Toast.makeText(this, "接口错误,请稍后重试", Toast.LENGTH_SHORT).show();
}
});
}
}
参数说明
模块配置的参数是OpenFeedParams,BlockParamsBuilder完成相关配置
OpenFeedParams params = new OpenFeedParams.BlockParamsBuilder()
.feedStyle(feedStyle) // 仅支持FeedStyle.DOUBLE_LIST_D
.setEnableMoreLiveEntry(true) // 是否显示更多直播入口,默认显示
.setFeedTitle("抖音直播") // 配置直播标题
.build();
三、关注天窗
使用场景
关注天窗是横向的列表,可嵌入页面中任何位置。
示意图
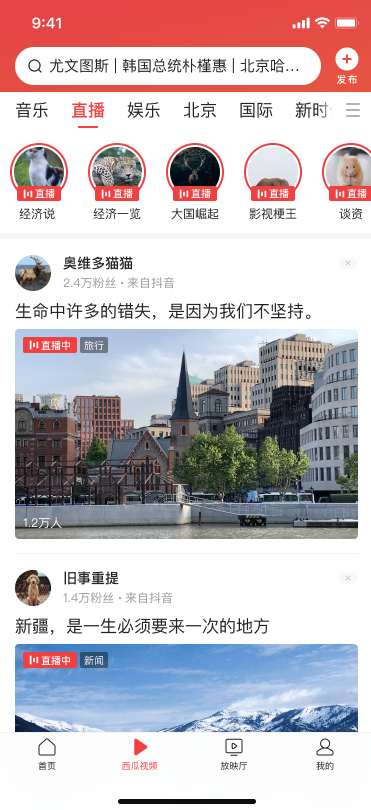
接入方式
通过IOpenLiveListFactory#createHorizontalLiveBlock获取关注天窗的view。
// 1. 获取IOpenLiveListFactory
private val liveListFactory: IOpenLiveListFactory? by lazy {
TTLiveService.getLiveService()?.openLiveListFactory
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activty_feed_test)
liveListFactory?.let {
horizontal_list_container_follow.removeAllViews()
// 2. 配置参数
val followFeedParams = HorFollowListParams.Builder()
.backgroundColor(Color.WHITE)
.build()
// 3. 获取关注天窗的view,ILiveBlock是View
followFeedHorizontalListView = it.createHorizontalLiveBlock(thi
s, followFeedParams)
horizontal_list_container_follow.addView(followFeedHorizontalListView as View)
// 4. 调用refresh获取数据
followFeedHorizontalListView?.refresh()
// 5. 监听列表状态,列表为空是隐藏容器
followFeedHorizontalListView?.isEmpty()?.observe(this, Observer { isEmpty ->
when (isEmpty) {
true -> horizontal_list_container_follow.visibility = View.GONE
false -> horizontal_list_container_follow.visibility = View.VISIBLE
}
})
// 6. 监听列表状态,接口错误时隐藏容器
followFeedHorizontalListView?.isError()?.observe(this, Observer { isError ->
when (isError) {
true -> horizontal_list_container_follow.visibility = View.GONE
false -> horizontal_list_container_follow.visibility = View.VISIBLE
}
})
}
}
参数说明
HorFollowListParams-可配置部分UI
val followFeedParams = HorFollowListParams.Builder()
.backgroundColor(Color.WHITE) // 关注天窗背景色
.avatarBorderAnimController(customAnimController) // 关注天窗的头像和头像边框动画
.liveTagColor(Color.BLACK) // 直播中标签颜色
.liveTagRadius(ResUtil.dip2px(3)) // 直播中标签圆角
.build()